In this C++ practice section you will get the basic idea about C++ real life problem solving.
We will discuss the solution of some basic real life problems here.
By solving them you can start your problem solving journey to any competitive programming platforms.
You should have believe that every problem has several solutions and here we will give you the most probable solutions with the description.
Your learning depends on your practice. The more you practice, the more you learn as well as the more mistake you will do.
So, never worry about your mistakes. Try to solve again and again with new idea.
However, you should see our C practice too and try to implement your own logic by C++ to those problems.
If you have successfully completed our C and C++ practice section, then you are ready to solve problem in any competitive programming websites.
Table of Contents
ToggleTurbo sort problem solving by C++
Read the description of turbo sort problem carefully before try to solve it.
Problem description
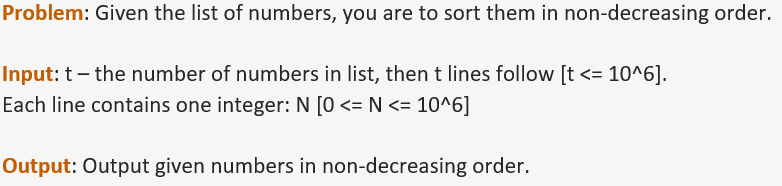

This turbo sort problem is all about sorting a list of numbers. The user will give some numbers and we have to sort them in non-decreasing order.
we can write the solution of this problem in different way and here we will see the probably best way to solve this problem.
The problem is taken from CodeChef for practice and learning purpose only.
At first we will try to understand about the problem and go to code. So, let’s go ahead with coding.
Solution of turbo sort problem using C++
At first we will take the input from user that how many numbers he has for sorting.
We will store all the numbers in an array and then we will apply a library function to sort them.
This is very simple to use the sort() function which will take two parameters.
One is the beginning of the array and other is the length up to which we want to sort.
If a user gives the number of total number is 4.
Then 4 number is 5, 7, 3, 2
We have to sort them and print 2, 3, 5, 7
Here, we have to print each of them in a new line.
Now, let’s see the source code for turbo sort problem bellow;
// solving turbo sort problem
#include<iostream>
#include<bits/stdc++.h>
using namespace std;
int main(){
int Test;
scanf("%d", &Test);
int arr[Test];
for(int i = 0; i < Test; i++){
scanf("%d", &arr[i]);
}
sort(arr, arr+Test);
for(int i = 0; i < Test; i++){
printf("%d\n", arr[i]);
}
return 0;
}
When you compile and run the above program, you will get the output like this.
Output of turbo sort problem
5
5
3
6
7
1
1
3
5
6
7
In this output screen, first line contains the number of total number which is 5. Then 5 line and each line has a single integer which we have to sort.
The last five lines is the output of our program after sorting given numbers.
Small factorials problem solving by C++
Read the description of small factorials problem.
Problem description


Here in this small factorials problem we have to calculate factorial of a number.
The user will given t numbers and we have to calculate the factorial of all these number where t is the testcase.
You may have think that we can do it very simply.
But if you want to find the factorial of comparatively large number such as 70, then you will find that then result number is so big that we can not store it into any variable.
For this we have take an array to store such big number. However, this problem is taken from CodeChef for practice and learning purpose only.
Here we will discuss the solution of this problem and see the code in C++.
Solution of small factorials problem
Suppose, you want to determine the factorial of 5.
Then 5! = 5 * 4 * 3 * 2
= 120
This is quite easy to determine. But if you think about the number 70.
Then 70! =11978571669969891796072783721689098736458938142546425857555362864628009582789845319680000000000000000
Can you imagine the number? This is a very big number and we can not store it into any variable.
But if we take an array to store this number then the problem will be solved.
Here in the bellow program we have implemented the logic to store the number inside an array.
We will multiply the result one by one and store the result to the array. Let’s see the program bellow.
// solving small factorial problem
#include<iostream>
using namespace std;
int main(){
int t;
cin >> t;
for(int i = 0; i < t; i++){
int n;
cin >> n;
int size = 1000, nfact[size], carry = 0, j = size-1;
nfact[size - 1] = 1;
while(n > 1){
int x;
for(int k = size-1; k >= j; k--){
x = nfact[k]*n + carry;
nfact[k] = x % 10;
carry = x / 10;
}
while(carry > 0){
nfact[--j] = carry % 10;
carry /= 10;
}
n--;
}
for(int k = j; k < size; k++){
cout << nfact[k];
}
cout << endl;
}
return 0;
}
Output of this small factorials problem is given bellow;
Output of small factorial problem
4
1
1
2
2
5
120
3
6
First line of the output contains 4 which means we have to calculate the factorial of 4 numbers.
Then each line contains an input and the bellow line gives the output. The factorial of 1 is 1. Similarly, 2! = 2, 5! = 120 and 3! = 6.
ATM problem and solution by C++
Read the description of ATM problem bellow;
Problem description
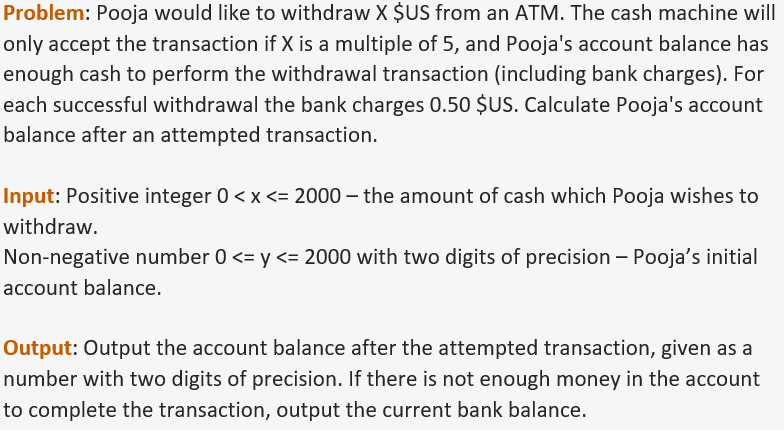

In this ATM program we have to calculate the remaining balance after any transaction.
If the balance is sufficient for transaction, we have to print the remaining balance after transaction.
But if the balance is insufficient, then we have to print the current balance.
There is also a condition in this ATM program that the ATM machine receives only the withdrawal request of the multiple of 5$.
If the user places the withdrawal request which is not multiple of 5 then we have to print the current balance as the withdrawal is not successful.
This problem is taken from CodeChef for practice and learning purpose of our readers.
In this C++ practice article we will discuss about the solution of this problem with source code.
Solution of ATM problem
We will take two input from the user which will be the amount the user want to withdraw and the current balance of his account.
As the bank charges 0.50$ for each successful transaction. We have to consider the total balance is sufficient for withdraw with bank charges.
Imagine, the user gives two number 30 and 120.00.
Then we have to print the remaining balance is
120.00 – (30 + 0.50)
= 89.50
But if the user gives two number such as 300 and 120.00.
Then it is not possible to withdraw the money as the remaining balance is not sufficient for this withdrawal request.
Again, any withdrawal request which is not the multiple of 5, will not be successful. Then we have to print the current balance also.
Now, let’s see the source code for atm problem solving in C++.
// solving atm problem by c++
#include <iostream>
#include <iomanip>
using namespace std;
int main(){
int tran;
float newBal;
cin >> tran >> newBal;
if(tran % 5 == 0 && newBal >= (tran + 0.5)){
newBal = newBal - (tran + 0.5);
}
cout << fixed << setprecision(2) << newBal << endl;
}
Try to give different input to test the program. You will see the output like this.
Output of ATM problem
30 120.00
89.50
42 120.00
120.00
300 120.00
120.00
For each case in output console, the first number is the amount which the user want to withdraw.
The second number is the current balance. Second line contains the output for specific input.
Find reminder problem solution in C++
Read the description of find reminder problem carefully;
Problem description
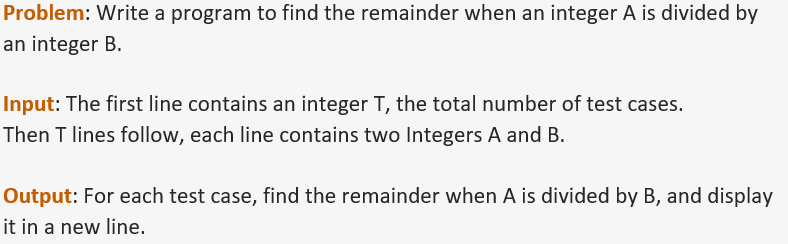

In this find reminder simple problem we have to find the reminder dividing A by B.
The number A and B is given by the user. We have to store the given number into two variables and the calculate the reminder of A/B.
This is a very simple problem and if you have completed our C++ programming section, then it will be very simple to solve for you.
However, this problem is taken from CodeChef for practice and learning purpose of our readers.
In this article we will solve the problem and discuss how the program works. So, let’s go ahead.
Solution and source code of find reminder problem
We will take the number of test case from the user and then take two input for every test case.
We will store that two input in variable a and b. The we will find the reminder using a % b and print that reminder.
Consider, the user gives two integer 10 and 3. Then we have to print the reminder of dividing 10 by 3. We know the reminder will be 1 for these two inputs.
10 % 3 = 1;
Now, we will see the source code to implement this logic for printing the reminder.
// solving find reminder problem using C++
#include<iostream>
using namespace std;
int main(){
int t, rem = 0;
cin >> t;
for(int i = 0; i < t; i++){
int a, b;
cin >> a >> b;
rem = a % b;
cout << rem << endl;
rem = 0;
}
}
The output of this program will be as like as bellow.
Output of find reminder problem
3
1 2
1
100 200
100
40 15
10
First line contains the user input which is the test case for this problem. Then each test case takes two input a and b.
Then the third line is the output for the input of second line.
Sum of digits problem and solution in C++
Read the problem description of sum of digits problem here;
Problem description

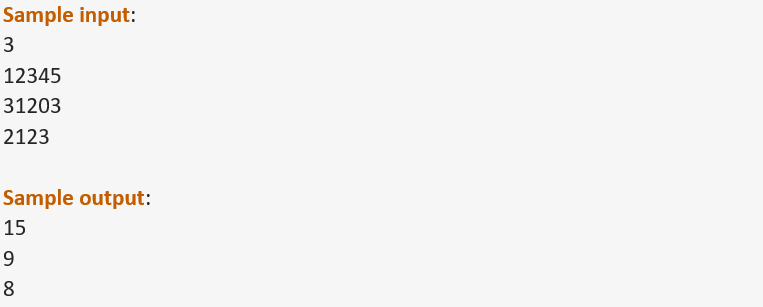
In this sum of digits problem we have to find the sum of all the digits of an integer given by the user.
We already have discussed about determining sum of digits in our C++ programming section. You can take the idea to solve this problem from there.
However, in this guide we will see the solution of this sum of digits problem. We have taken this problem from CodeChef for practice and learning purpose only.
After taking the number form the user we will calculate the the sum of digits of that number here.
Solution of sum of digits problem in C++
For determining the sum we will take the input first which will be the test case of this program.
Then each test case will take a number and calculate the sum of digits like bellow;
Assume, the user gives 5634 to find the sum of digits. The program will calculate,
(5 + 6 + 3 + 4) = 18
We can implement the logic to find the sum in different way which we have discussed in our sum of digits in C and sum of digits in C++ articles.
If you have successfully completed those two guide then this program will be very simple to solve for you.
Now, let’s see the source code to find the sum of digits in C++ bellow.
// sum of digits problem solving in C++
#include <iostream>
using namespace std;
int main(){
int t, sum = 0;
cin >> t;
for(int i = 1; i <= t; i++){
int num;
cin >> num;
while(num > 0){
sum += num % 10;
num /= 10;
}
cout << sum << endl;
sum = 0;
}
}
Compile and run the above program in your editor to get the output like this.
Output of sum of digits problem
3
12345
15
31203
9
2123
8
- Here, the first line contains the test case.
- Second line contains the number for first test case of which we have to calculate the sum of digits.
- Third line is the output for the first test case which have printed 15.
- Because (1 + 2 + 3 + 4 + 5 = 15).
- Similar process for other test case also.
Enormous input test problem solving by C++
Read the problem description of enormous input test problem here;
Problem description
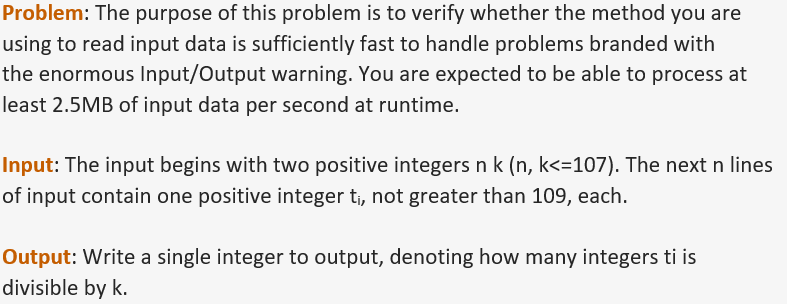

In this enormous input test problem we have to test how many given numbers are divisible by a specific number.
The user will give two integer first. The first number is the number of integers which we have to check.
Second integer is that specific integer by which we have to check the divisibility of the upcoming integers.
After checking all the integers we have to print how many integers are divisible by that number.
We have taken this enormous input test problem from CodeChef for practice and learning purpose. We will discuss about the problem and its solution in this guide.
Solution of enormous input test problem
Imagine the user gives two number which is 7 and 3. Now, the user will give more 7 integers and we have to check how many integers among them are divisible by 3.
Consider he has given the more 7 integers which are as follows;
3
55
966
9
2
999
10
Now, we will check which integers are divisible by 3. Here, 3, 966, 9 and 999 are divisible by 3. As total 4 integers are divisible by 3 and so we will print the output 4.
Let’s go the the source code of enormous input test bellow;
// enormous input test problem solving in C++
#include<iostream>
using namespace std;
int main(){
int i, j, counter = 0;
cin >> i >> j;
for(int x = 0; x < i; x++){
int y;
cin >> y;
if(y % j == 0){
counter++;
}
}
cout << counter << endl;
return 0;
}
Compile and run this enormous input test program to get the following output.
Output of enormous input test problem
7 3
1
51
966369
7
9
999996
11
4
In the output console first line contains the two number. First is the number of upcoming integers which we have to test.
Second one is the number by which we will divide the upcoming integers. Then 7 line and each line has one integers.
At the last line we have printed our output which is 4. Because only 4 integers are divisible by 3 here.
Thanks for finally writing about > C++ Practice Problem | Real Life Problem Solving With C++
– World Tech Journal < Liked it!