In this article we will see 4 different C programs to check vowels of English Language. We will check whether a given character is vowel or consonant.
It is well known that the letter “a, e, i, o, u” in both the uppercase and lowercase are considered as vowel.
First we will take a character input from user and then check it that the user has given a vowel or consonant. We can do this by several logic. Let’s see some of them bellow.
Table of Contents
ToggleC programs to check vowels using if else condition
If else statement is one of the most uses control flow statement in not only C programming but also any other programming languages.
You can apply different logic to check a character that it is vowel or consonant by if else statement. Let’s see a very simple logic among them.
#include <stdio.h>
int main(){
char myChar;
printf("Enter a character to check : ");
scanf("%c", &myChar);
if(myChar == 'a' || myChar == 'e' || myChar == 'i' || myChar == 'o' || myChar == 'u' ||
myChar == 'A' || myChar == 'E' || myChar == 'I' || myChar == 'O' || myChar == 'u'){
printf("\n\t%c is vowel.\n", myChar);
}else{
printf("\n\t%c is consonant.\n", myChar);
}
return 0;
}
The program given above is not well organized although it is the simplest way. We can also write this program more efficiently as following.
#include <stdio.h>
int main(){
char myChar;
printf("Input a character to check : ");
scanf("%c", &myChar);
if ((myChar >= 'a' && myChar <= 'z') || (myChar >= 'A' && myChar <= 'Z')) {
if (myChar =='a' || myChar =='A' || myChar =='e' || myChar=='E' || myChar=='i' || myChar=='I'
|| myChar=='o' || myChar=='O' || myChar== 'u' || myChar=='U'){
printf("\n%c is a vowel.\n", myChar);
}else{
printf("\n%c is a consonant.\n", myChar);
}
}else{
printf("\n%c is neither a consonant nor a vowel.\n", myChar);
}
return 0;
}
Output of this C program to check vowels
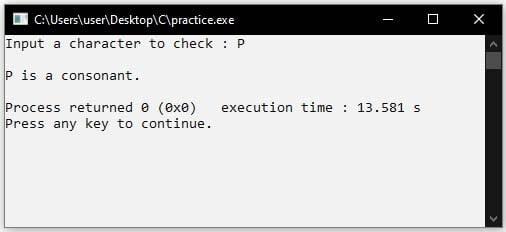
C programs to check vowels using switch statement
Switch statement is also a control flow statement in C programming. Here we can use a switch statement to check vowels.
Here is the program to check vowels using switch statement in C language.
#include <stdio.h>
int main(){
char myChar;
printf("Input a character here : ");
scanf("%c", &myChar);
switch(myChar){ // switch statement
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
case 'A':
case 'E':
case 'I':
case 'O':
case 'U':
printf("\n%c is a vowel.\n", myChar);
break;
default: // It may be vowel or other character
printf("\n%c is not a vowel.\n", myChar);
}
return 0;
}
Output:
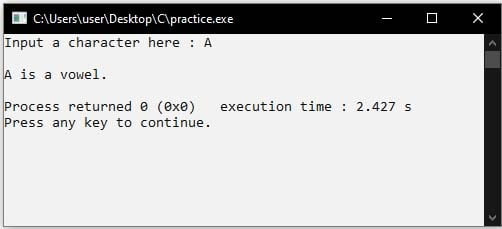
C programs to check vowels using function
Both the built-in function and user defined functions has a significant role in C programming.
It is always recommended to use function to make the code efficient as we can use a function from different levels of a program.
Here is the program to check vowels using a switch statement in C language.
// C programs to check vowels using function
#include <stdio.h>
int isVowel(char x){ // function to check vowel
if (x == 'A' || x == 'E' || x == 'I' || x == 'O' || x == 'U'){
return 1;
}else if (x == 'a' || x == 'e' || x == 'i' || x == 'o' || x == 'u'){
return 1;
}else{
return 0;
}
}
int main(){
char x;
printf("Enter the character you want to check : ");
scanf("%c", &x);
if(isVowel(x)){ // calling function inside if condition
printf("\nVowel\n");
}else{
printf("\nNot a vowel\n");
}
return 0;
}
Output of this check vowel program
Enter the character you want to check : u
Vowel
Process returned 0 (0x0) execution time : 5.044 s
Press any key to continue.
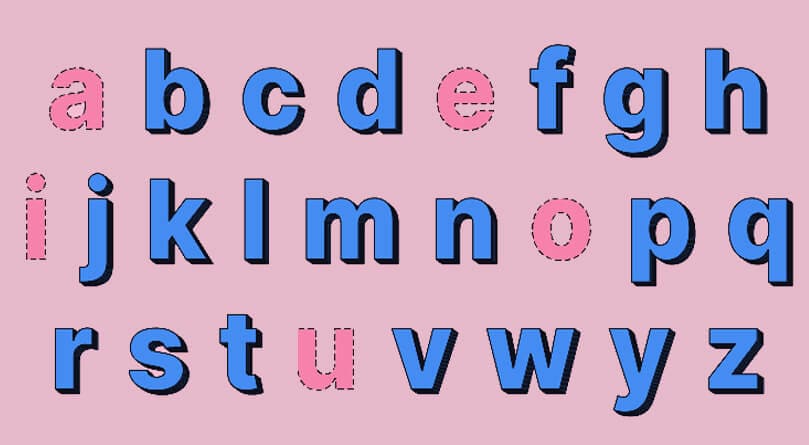
C programs to check vowels have a great importance if you are beginning at C programming.
In this above guide, I hope you are now clear about how to check an alphabet that it is vowel or consonant.
The comment section is open for all to share your opinion or experience about the discussed topics here.